Okay, so I was messing around with this idea for a crossword puzzle app, and I thought, “Why not document the whole messy process?” So, here’s how it all went down, from a pretty vague idea to something… well, slightly less vague.
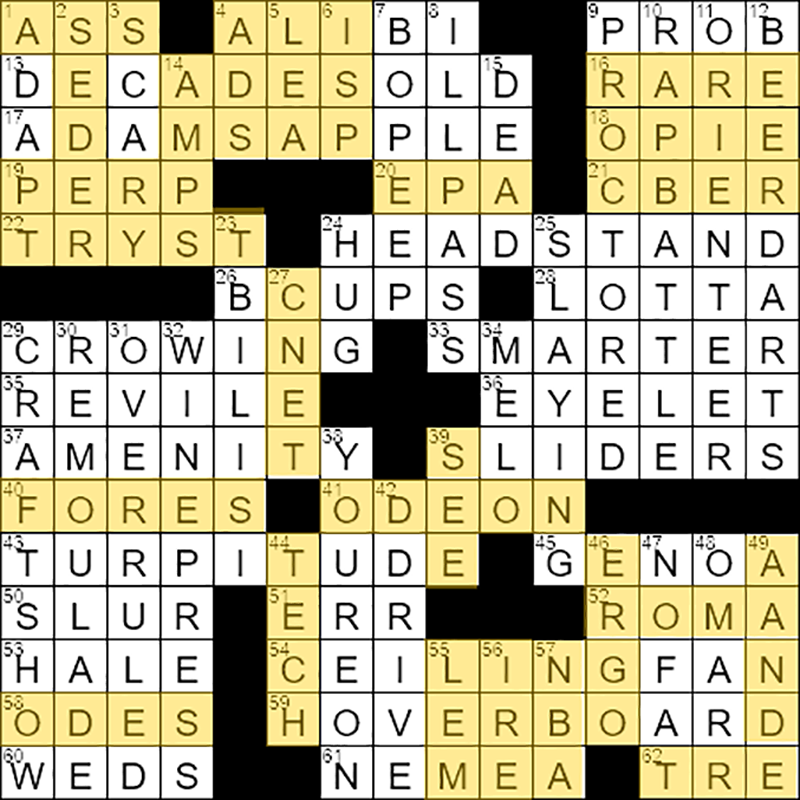
Brainstorming and Initial Setup
First, I grabbed a pen and paper – yeah, old school, I know – and started jotting down anything that came to mind about crossword suggestions. I figured I needed a way to:
- Store a bunch of words and their clues.
- Search through them quickly.
- Filter based on, like, the letters already in the grid.
I decided to use Python because, well, it’s what I’m most comfortable with. I created a new file and just started coding, no fancy planning or anything. My initial thought was to just use a simple list of dictionaries, each dictionary holding a word and its clue.
like this:
word_list= [
{"word":"EXAMPLE","clue":"A sample word"},
{"word":"SUGGEST","clue":"Put an idea forward"}
The First (Ugly) Function
I whipped up a basic function to search through this list. It was pretty clunky. I used some basic string matching, nothing too clever. Basically, it just checked if the search term was in the clue. and words.
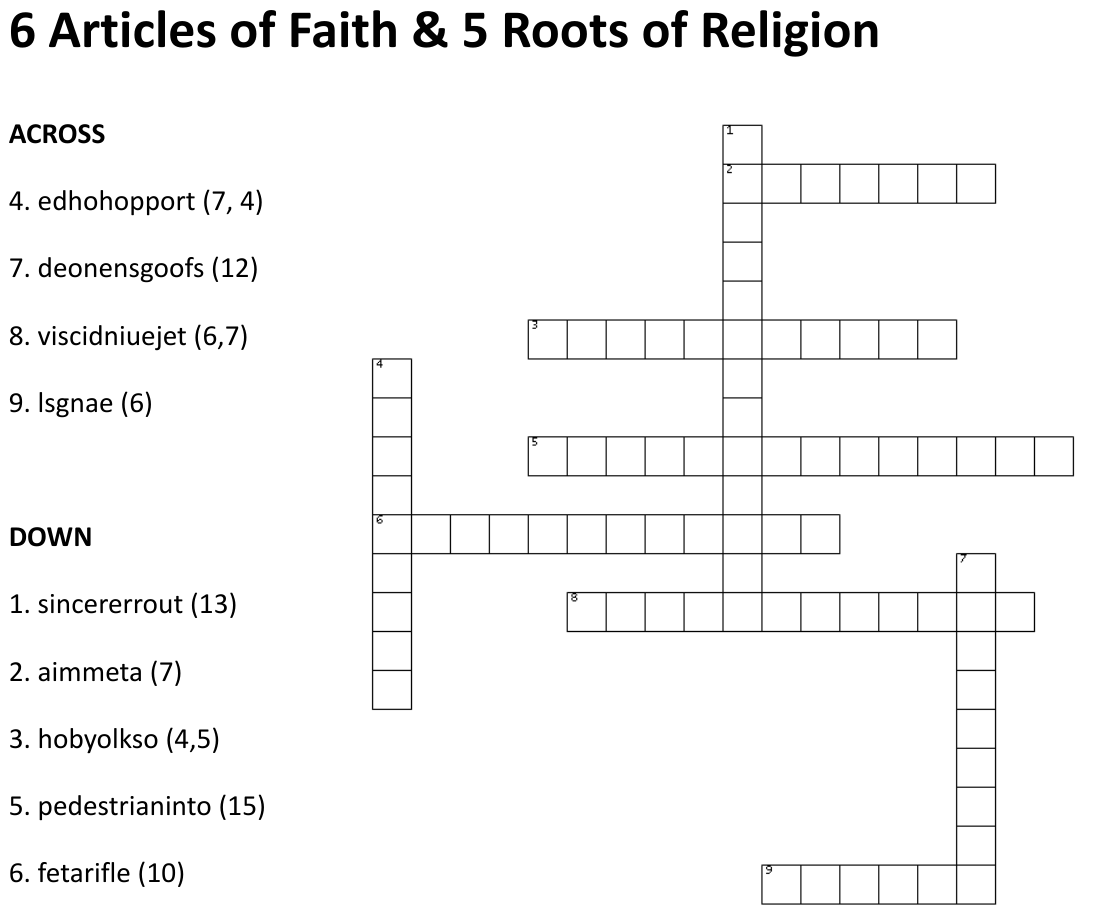
Trying to Make it Smarter
Then, I realized I needed a way to handle the “fitting” part – you know, like when you have “A?PLE” and you need a five-letter word starting with “A” and having “P”, “L”, and “E” in the right spots.
So, I added another function. This one was even messier. It took the partially filled word (like “A?PLE”) and the word list, and it tried to find words that matched the pattern. I used a bunch of `if` statements and string slicing. It was… not pretty. But it kinda worked! It would correctly suggest the “APPLE”.
Realizing the Limitations
After playing around with it for a while, I started to see some big problems. My simple list-based approach was going to be way too slow for a large number of words. Plus, my matching logic was super basic and wouldn’t handle anything slightly complex.
Thinking About Better Solutions
I spent some time thinking about how to make it better. I figured I probably needed a more efficient data structure, maybe some kind of tree or something. I also thought about using regular expressions for more powerful pattern matching.
Where I Left Off
Honestly, I haven’t gotten around to implementing any of those fancier ideas yet. It’s still in that “proof-of-concept” stage, where it’s more “proof” than “concept.” But hey, it was a fun little exercise, and I learned a few things along the way. Maybe I’ll revisit it someday and turn it into something actually useful. For now, it’s just a bunch of messy code that kinda-sorta suggests crossword answers.