Okay, so I was messing around with Astro the other day, and I had this idea for a cool-looking “ring” component. I figured I’d walk you through how I built it, ’cause it’s kinda neat and might give you some ideas for your own projects.
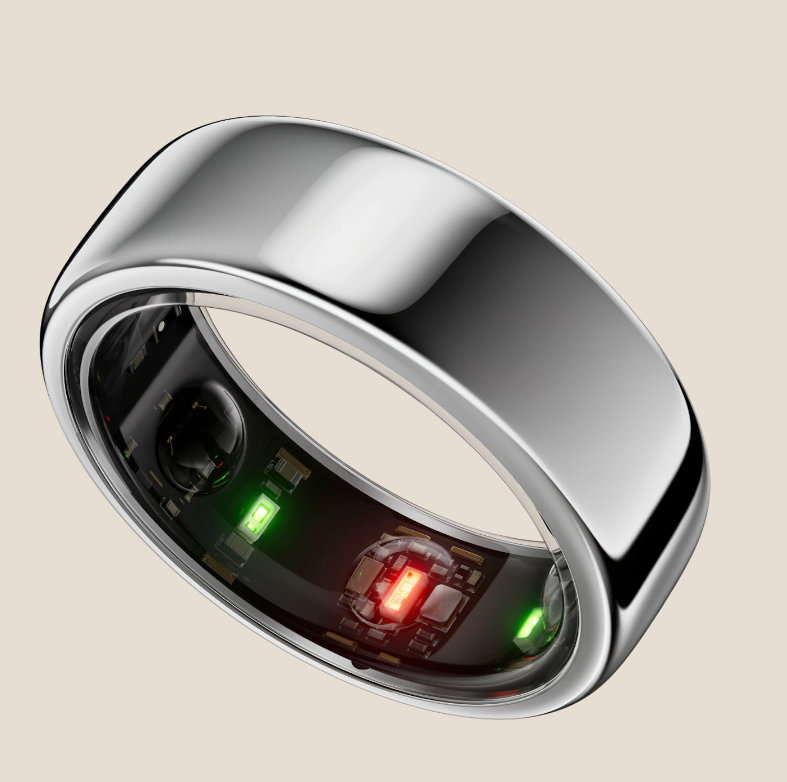
Getting Started
First, I spun up a new Astro project. Nothing fancy, just the basics. You know, the usual npm create astro@latest
and follow the prompts. I opted for the “empty” template, ’cause I like to build things up from scratch.
Building the Ring
So, the core idea was to use CSS to create the ring shape. I decided to go with a simple circular div and then use borders and shadows to get that “ring” effect. I created a new component file called .
Inside , I started with a basic div:
astro
Pretty straightforward, right? Now for the CSS. I added a <style>
block within the component to keep things contained:
astro
.ring {
width: 100px;
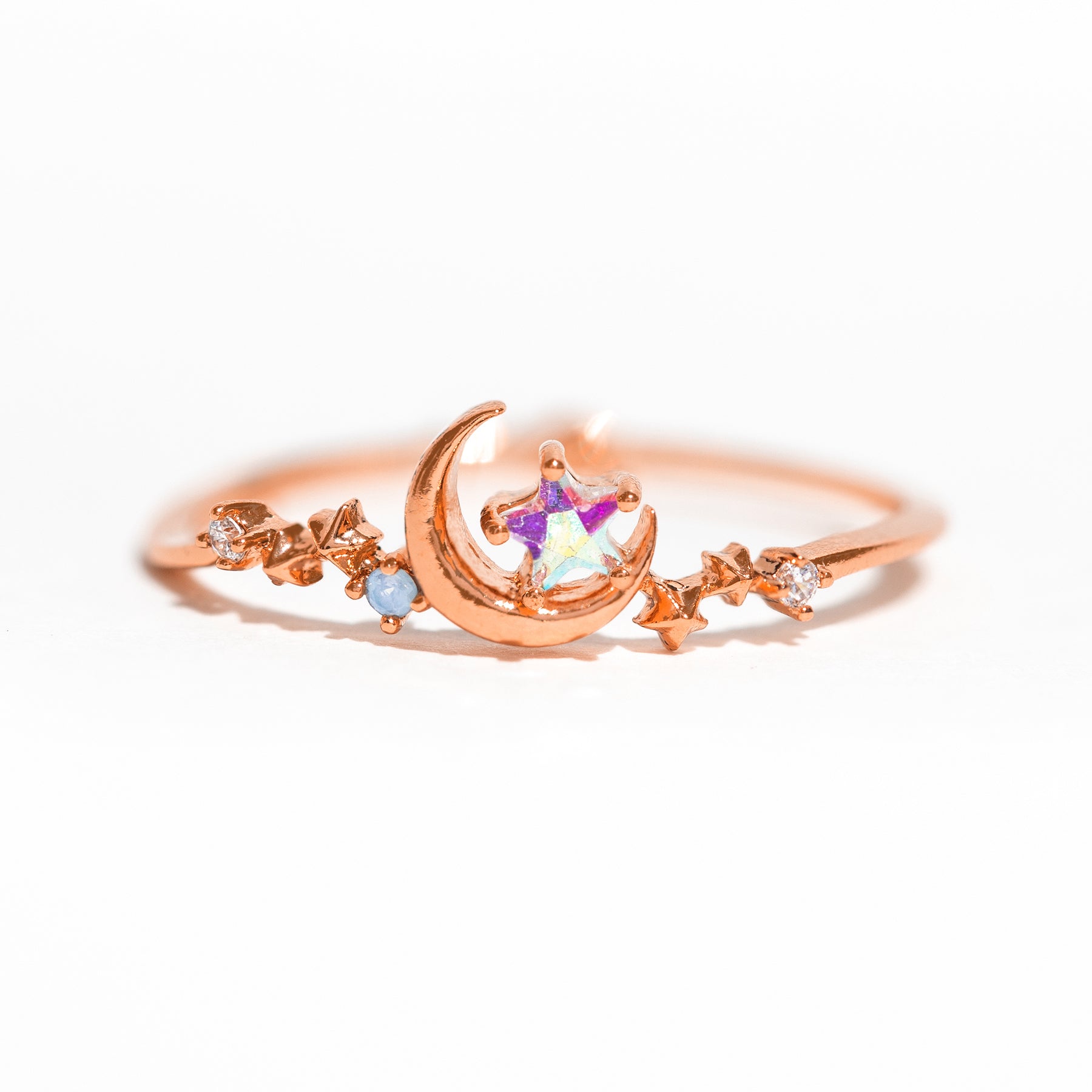
height: 100px;
border-radius: 50%; / Makes it a circle /
border: 5px solid #ccc; / The outer ring /
box-shadow: 0 0 10px rgba(0, 0, 0, 0.5); / A subtle shadow /
I played around with the width
, height
, border
width, and box-shadow
values until I got something I liked. It’s all about tweaking those numbers to get the look you’re after.
Adding Some Polish
To make it a bit more interesting, I wanted to add an inner ring. I did this by adding another border
with a different color and a slightly smaller width, using the `inset` in the `box-shadow`.
Here’s the updated CSS:
astro
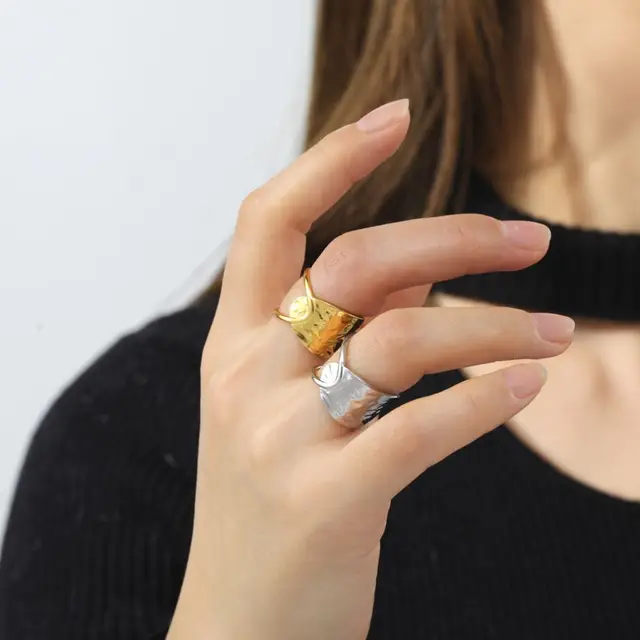
.ring {
width: 100px;
height: 100px;
border-radius: 50%;
border: 5px solid #ccc;
box-shadow:
0 0 10px rgba(0, 0, 0, 0.5),
inset 0 0 5px rgba(115, 19, 181, 0.8);
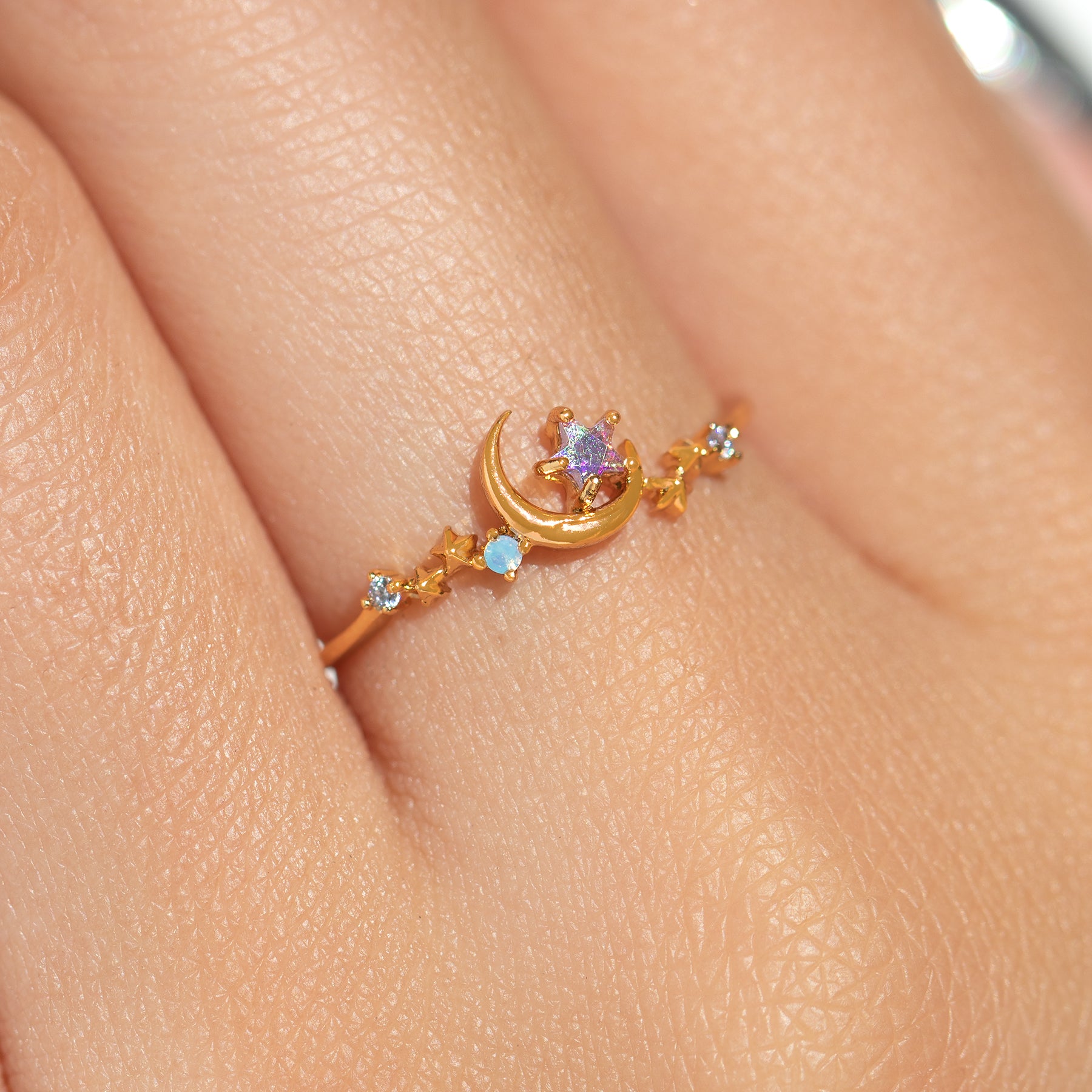
I decided that to give my ring more life, I would add an animation to it.
So with that, I’m going to add some animation using keyframes.
astro
.ring {
width: 100px;
height: 100px;
border-radius: 50%;
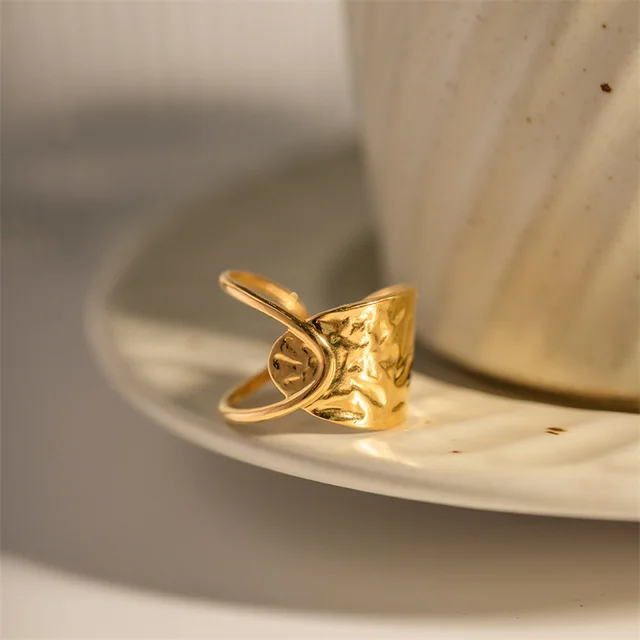
border: 5px solid #ccc;
box-shadow:
0 0 10px rgba(0, 0, 0, 0.5),
inset 0 0 5px rgba(115, 19, 181, 0.8);
animation: rotate 2s linear infinite;
@keyframes rotate {
from {
transform: rotate(0deg);
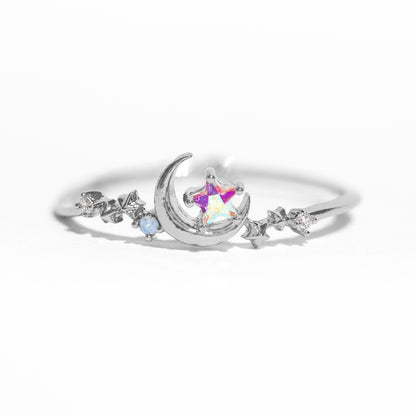
to{
transform:rotate(360deg);
Using the Component
Now, to actually use the ring, I just imported it into my page (or whatever your main page is) and dropped it in:
astro
import Ring from ‘../components/*’;
And there you have it! A simple, reusable ring component built with Astro and CSS. You could easily extend this by adding props to control the size, colors, or even the animation. Go wild with it!