Alright, let me tell you about this little project I did called “league of legends ban check.” It’s nothing fancy, but it saved me and my buddies a whole lot of headache when trying to play together.
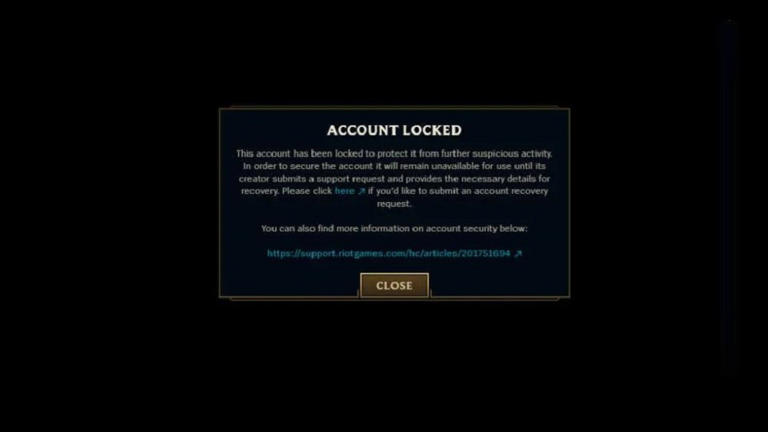
So, it all started like this: me and my friends, we’re all about that League life, right? But there’s always that one guy, or maybe even two, who are constantly getting chat restricted or straight-up banned. Trying to figure out if everyone’s good to queue up was always a “hold on, let me check” kinda deal. Super annoying, especially when you just wanna jump into a game.
I was like, “There’s gotta be a better way.” I mean, I mess around with Python every now and then, so I figured I could probably hack something together.
First thing I did was dive into Riot’s API. Getting a dev key was surprisingly easy. Then, I started messing around with the API endpoints to see what kinda info I could pull. Turns out, you can actually check a summoner’s ban status, but it’s not as straightforward as you might think.
The core idea was this: I needed a way to input a list of summoner names (that’s the in-game name, if you’re not a LoL player). Then, for each name, I’d hit the Riot API, grab the summoner ID, and then use that ID to check for any active restrictions.
Here’s the basic flow I ended up with:
- Take summoner names as input (I started with just pasting them into the script).
- For each name:
- Call the Riot API to get the summoner ID.
- Call the Riot API again, this time using the ID, to check for restrictions.
- Print out the summoner name and their ban status (“Clean,” “Chat Restricted,” “Banned,” etc.).
Now, the actual code? It was a bit messy at first. Lots of trial and error. I was wrestling with the API responses, trying to parse the JSON, and handling potential errors (like when a summoner name doesn’t exist, or the API is down). I used the `requests` library for making the API calls and `json` for handling the data. Pretty standard stuff.
One tricky part was dealing with rate limits. Riot doesn’t want you spamming their API, so they put limits on how many requests you can make in a certain time period. I had to add some `*()` calls to slow things down a bit and avoid getting my API key temporarily blocked. It was a pain, but gotta play by the rules.
After a bunch of tweaking, I got a working script! It wasn’t pretty, but it did the job. I could paste in a list of summoner names, run the script, and quickly see who was good to go and who was stuck in the penalty box.
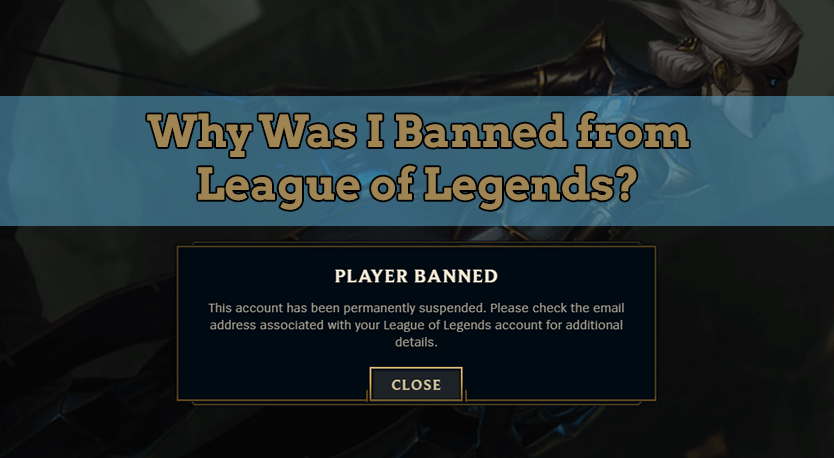
Of course, I wasn’t gonna stop there. I wanted to make it a little more user-friendly. So, I added a simple command-line interface using `argparse`. Now, I could just run the script with the summoner names as arguments, like this:
bash
python lol_ban_* SummonerName1 SummonerName2 SummonerName3
Way better than copy-pasting into the script itself!
The output wasn’t anything amazing, just plain text, but it was clear and easy to read. Something like:
SummonerName1: Clean
SummonerName2: Chat Restricted
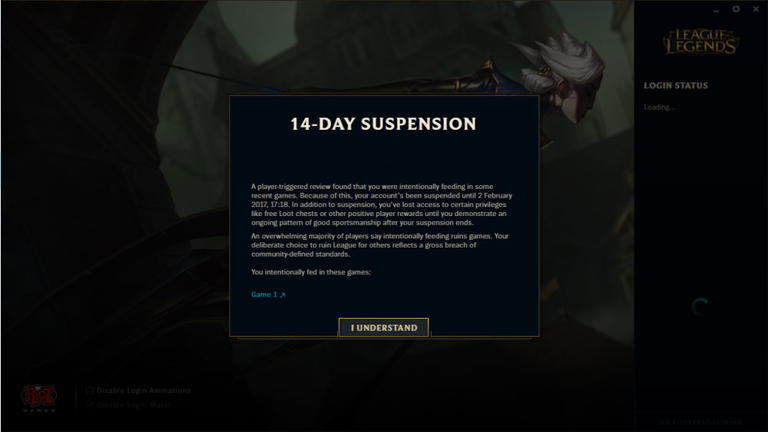
SummonerName3: Banned
That’s all I needed. We used it for a good while. It saved us a ton of time and frustration.
Looking back, it was a pretty simple project, but it was a good learning experience. I got more comfortable with using APIs, handling JSON data, and dealing with rate limits. Plus, it solved a real problem for me and my friends, which is always a good feeling.
I’m thinking about cleaning it up, maybe adding a GUI, and even hosting it online so anyone can use it. But for now, it does exactly what I need it to do. And that’s good enough for me.
Key takeaways:
- Riot API is pretty accessible.
- Rate limiting is a real thing; be mindful of it.
- Even simple scripts can be incredibly useful.
That’s the story of my “league of legends ban check” project. Hope you found it interesting!