Alright, so yesterday I was messing around trying to pull player stats from that Timberwolves vs. Nuggets game. Figured it’d be a fun little project to see how I could grab the data and maybe even do some basic analysis.
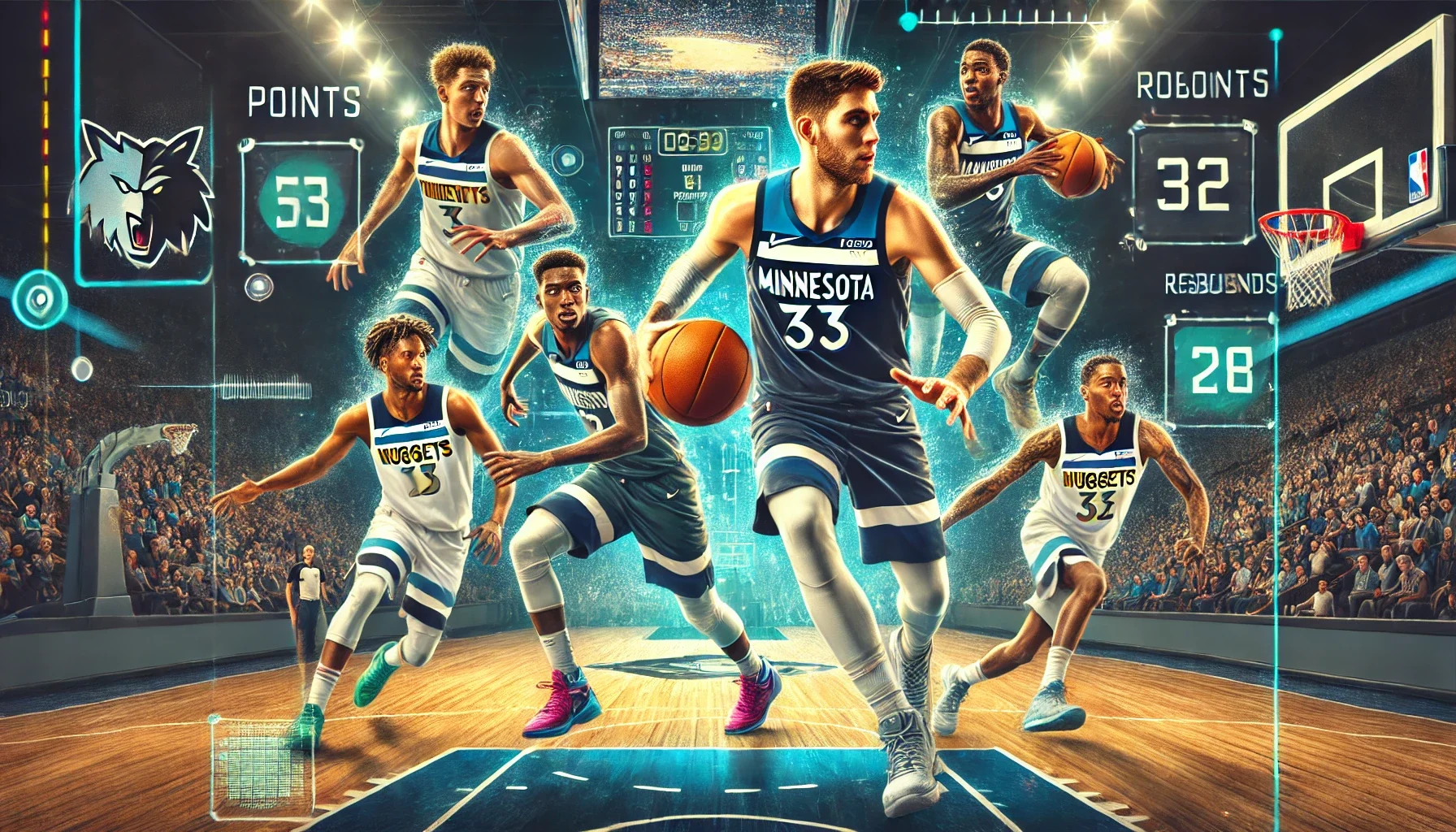
First thing I did was hunt down an API. I mean, scraping websites is a pain, right? I spent a good hour or so bouncing around different sports data APIs, some were pay-to-play, others were just… dead. Finally, I stumbled upon one that seemed to have what I needed – basic player stats for NBA games. It wasn’t perfect, the documentation was kinda sparse, but hey, beggars can’t be choosers.
Next up, whipped out Python. My go-to for this kinda stuff. I installed the requests
library because, well, gotta make those API calls somehow! Then I started writing some code to actually hit the API endpoint. This took a bit of trial and error, figuring out the right parameters to send to get the specific game data I wanted. Let me tell you, debugging API requests when the error messages are cryptic is a special kind of hell.
Once I got the data back, it was a giant JSON blob. Awesome! Now I had to parse that mess. I started by just printing out the raw JSON to the console to get a feel for the structure. Then, I used Python’s JSON library to load it into a dictionary. From there, I started digging through the nested dictionaries and lists to find the player stats. It was like navigating a maze, seriously.
The stats were there, but they weren’t exactly clean. Some players had missing data, others had weird formatting. So, I had to clean the data. This involved a bunch of loops and conditional statements to handle different edge cases. For example, some players didn’t have any assists, so the ‘assists’ field was null. Had to make sure my code didn’t choke on that.
After cleaning, I organized the data. I created a list of dictionaries, where each dictionary represented a player and their stats. Stuff like points, rebounds, assists, steals, blocks, you know, the usual. I also added a field for the team they played for, which was a bit tricky to extract from the original JSON.
Now that I had the data all nice and tidy, I wanted to actually do something with it. So, I did some basic analysis. Nothing fancy, just calculated the total points scored by each team and identified the top performers in different categories (most points, most rebounds, etc.). I just printed these results to the console, but you could easily save them to a file or visualize them with a library like Matplotlib.
Finally, I wrapped it all up in a function. This made it easy to reuse the code for other games. Just pass in the game ID and it spits out the player stats. I even added some error handling to catch common API errors and data cleaning issues.
Lessons learned? APIs are your friend, but documentation is often lacking. Data cleaning is always more time-consuming than you expect. And Python is a lifesaver for this kinda stuff. It was a fun little project and I now have a script I can reuse to pull NBA player stats whenever I feel like it. Maybe next I’ll try predicting the scores based on past performance… who knows!
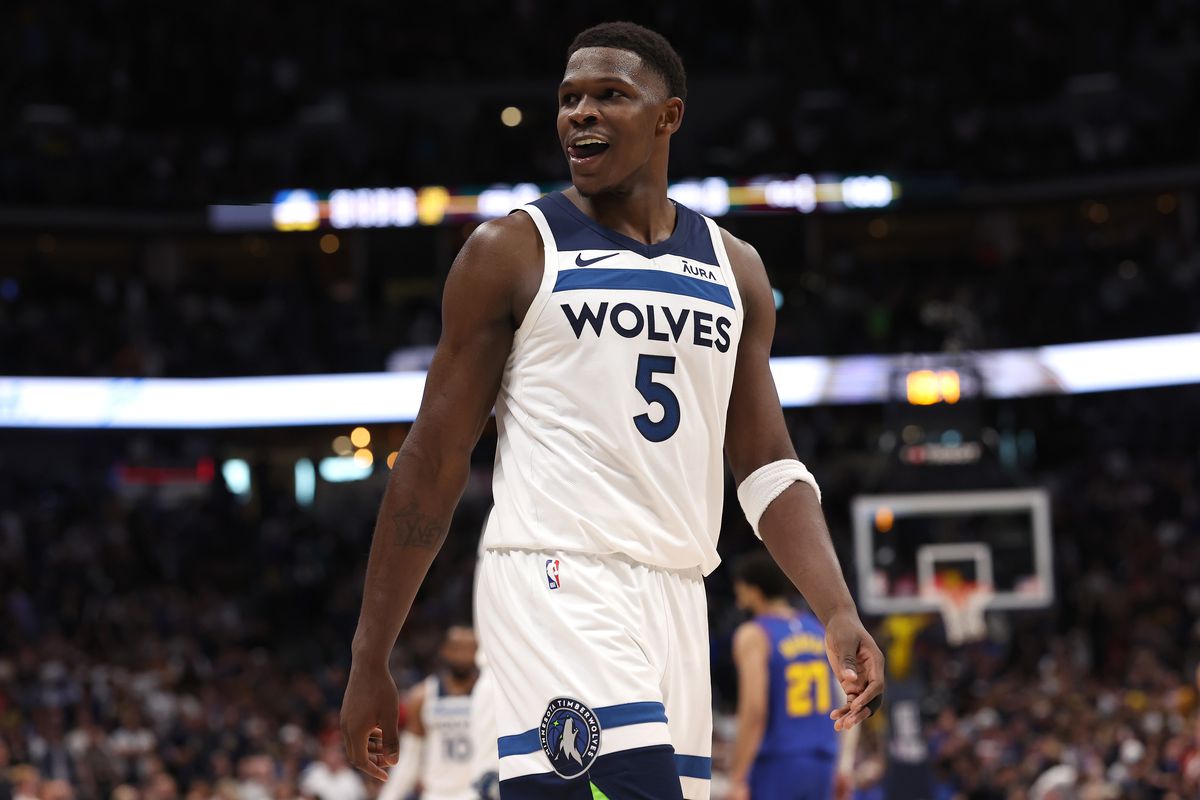