Okay, so yesterday I tackled a fun little project: solving a crossword puzzle using code. Sounds kinda nerdy, right? But trust me, it was a blast!
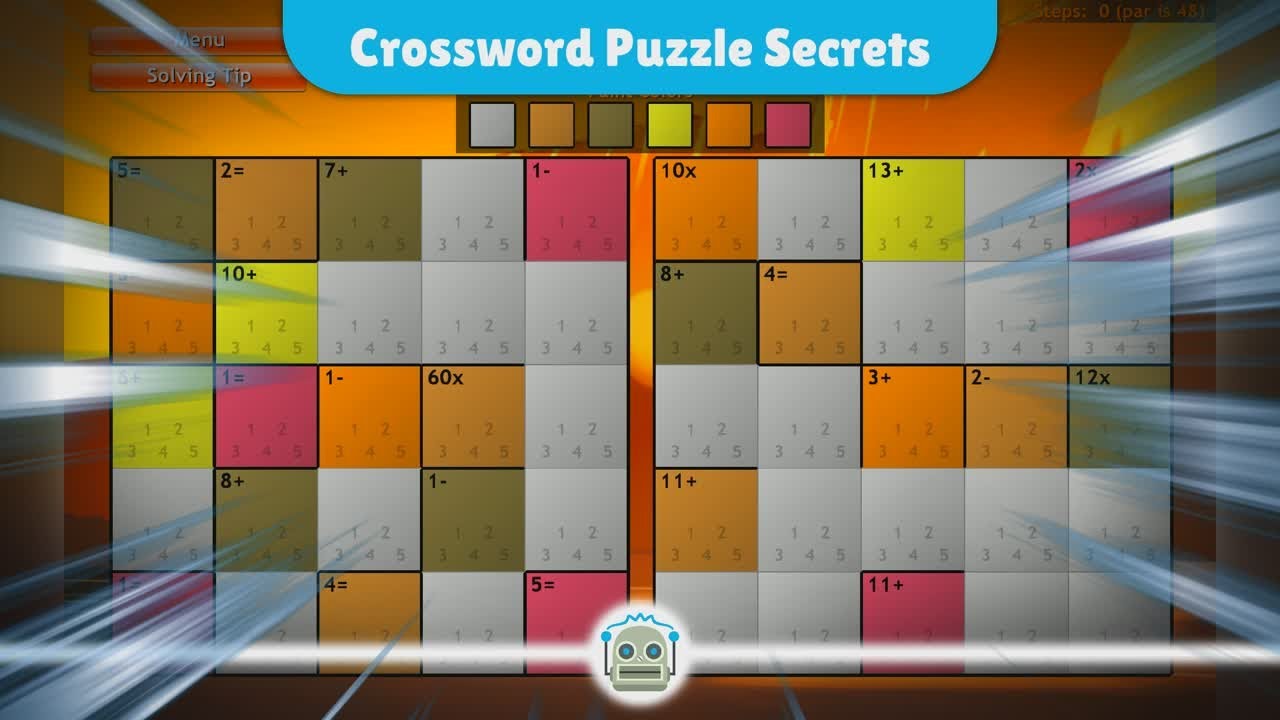
It all started with me stumbling upon a crossword in the newspaper. Usually, I just skim them, but this time, I thought, “Hey, could I automate this?” I mean, I’m a programmer, right? Let’s put those skills to the test.
First things first, I needed a plan. I figured I’d break it down into smaller steps:
- Get the crossword data: The clues and the grid structure.
- Find a word list: A massive dictionary to search through.
- Write a matching algorithm: Something to compare clues and possible words.
- Fill the grid: And hopefully, solve the darn thing!
I started with the crossword data. I didn’t want to manually type everything in, so I looked for an online version. Luckily, I found one! I copied the clues and sketched out the grid on a piece of paper. (Yeah, old school, I know.)
Next, the word list. I grabbed a giant word list (like, hundreds of thousands of words) from a public domain source. It was just a plain text file, one word per line. Perfect.
Now, for the tricky part: the matching algorithm. I thought about using regular expressions, but the clues were too vague for that. Instead, I decided to focus on two main things:
- Word length: The easiest filter.
- Known letters: If I already had a letter in a specific position, I’d use that to narrow down the possibilities.
I started writing some Python code. I created functions to:
- Read the word list into memory.
- Parse the crossword clues and grid layout.
- Filter the word list based on length.
- Check if a word matched any known letters in the grid.
The initial version was pretty basic. It would take a clue, find all words of the correct length, and then check if they matched any existing letters. It wasn’t great, but it was a start.
I quickly realized that the clues themselves needed some processing. They often contained synonyms or definitions. I tried using a simple thesaurus lookup, but that added a lot of complexity and didn’t always help.
So, I simplified things again. I decided to focus on clues that were more straightforward, like anagrams. I added a function to check if a word was an anagram of a part of the clue.
I ran the code on the crossword. It filled in a few words! Not a complete solution, but definitely progress. I tweaked the algorithm, added more filtering, and tried different approaches.
One thing that really helped was prioritizing the clues. I started by filling in the words with the fewest possible answers. That gave me more known letters to work with for the other clues.
After a few hours of coding and tweaking, I actually managed to solve a good chunk of the crossword! It wasn’t perfect, and I still had to fill in a few blanks manually, but it was way more successful than I expected.
The key takeaways for me were:
- Break down the problem into smaller, manageable steps.
- Start simple and iterate.
- Don’t be afraid to throw things away and try something new.
What I Learned
It was also a good reminder that even seemingly simple problems can be surprisingly complex. And that sometimes, the best approach is the simplest one.
It wasn’t a perfect solution, but it was a fun and challenging project. And who knows, maybe I’ll keep working on it and eventually build a fully automated crossword solver!
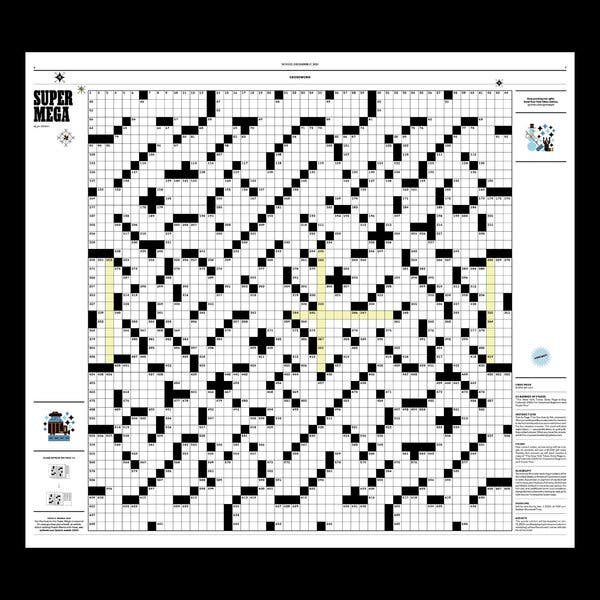
So, there you have it. My little experiment in crossword solving. It was a fun way to spend an afternoon, and I learned a lot in the process.