Alright, let’s dive into my sol3 phase 5 journey. Buckle up, it’s gonna be a bit of a ride.
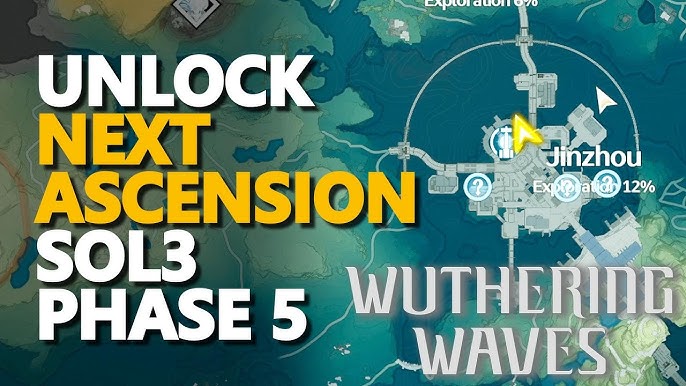
So, it all started when I decided, “Hey, why not try something new with sol3?” I’d been messing around with it for a while, doing the usual stuff – basic bindings, nothing too crazy. But I wanted to push it, really see what this thing could do.
First thing I did was sketch out a plan. I wanted to create a system where I could register C++ classes with Lua, and then have Lua scripts dynamically instantiate and manipulate those objects. Seemed simple enough, right? Hah!
I started by defining a base class in C++. Something super basic, like:
class BaseObject {
public:
virtual ~BaseObject() {}
virtual void print() { std::cout << "BaseObject::print()" << std::endl; }
Then, I moved on to binding it with sol3. This part was pretty straightforward, thanks to sol3’s relatively clean API. Used sol::usertype
and all that jazz.
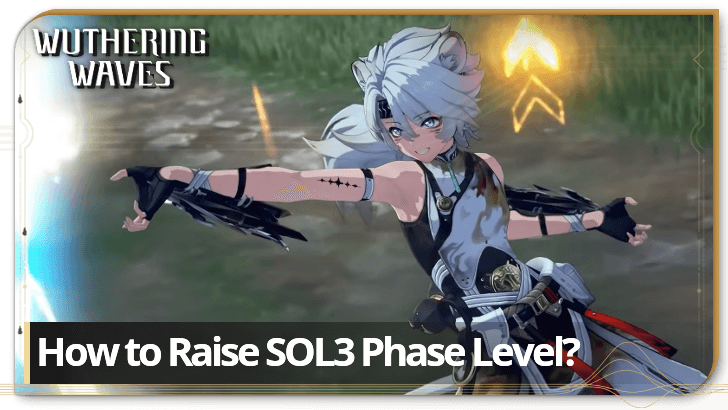
Next, I created a registration system in C++. This involved a bit of template magic. I needed a way to register derived classes, link them to Lua names, and create factory functions. It was messy, I’m not gonna lie. Lots of trial and error. I remember spending a whole afternoon debugging a stupid template instantiation issue. Ugh!
Then came the Lua side. I wrote a Lua script that would fetch the factory function for a registered class, instantiate it, and call its methods. The goal was to create a Lua script that could create C++ objects, which is where it gets interesting!
The biggest hurdle was memory management. Lua has its own garbage collector, and C++ has its own way of doing things. Making them play nice together was a challenge. I had to be super careful about who owns what, and when things get deleted. Spent a good chunk of time chasing down memory leaks, let me tell you.
After days of coding, debugging, and copious amounts of caffeine, I finally managed to get a basic version working. I could register classes, instantiate them from Lua, and call their methods. Victory! (Sort of.)
But it was slow. Like, REALLY slow. Every time I called a C++ method from Lua, there was a noticeable delay. I profiled the code and found that the bottleneck was in the sol3 library itself, specifically in the function call overhead.
So, I started digging into sol3’s internals. I tried different optimization techniques, like caching function pointers and reducing the number of copies. It helped a little, but not enough.
Eventually, I realized that I was fighting a losing battle. The overhead was inherent in the way sol3 was designed. It was never meant to be a super-high-performance solution. It was more about convenience and ease of use.
At that point, I decided to scale back my ambitions. I couldn’t get the performance I wanted without completely rewriting sol3, which was way beyond my capabilities (and my patience!). So, I focused on making the existing system as efficient as possible, and accepted that it would never be blazingly fast.
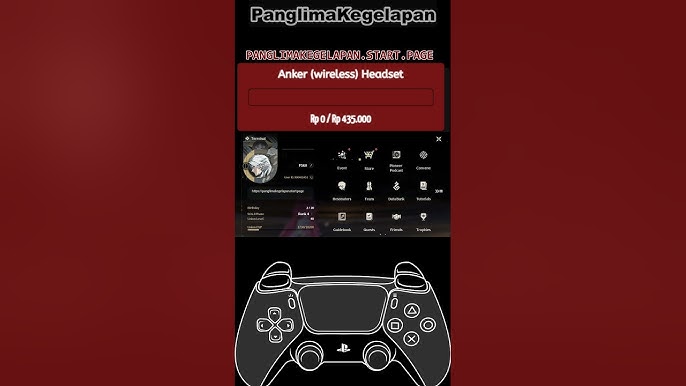
The final result? A system that allows me to dynamically create and manipulate C++ objects from Lua, but with a performance penalty. It’s not perfect, but it’s good enough for my purposes.
Lessons learned:
- Sol3 is great for simple bindings, but it has limitations when it comes to performance-critical applications.
- Memory management is a pain in the butt when you’re dealing with Lua and C++.
- Sometimes, you have to accept that you can’t solve every problem perfectly.
Would I do it again? Probably not. But I definitely learned a lot in the process. And that’s what matters, right?