Alright, so yesterday I decided to tackle something fun – building a crossword puzzle solver. I called it “crossword carefree” because, well, the goal was to make solving crosswords a bit more carefree.
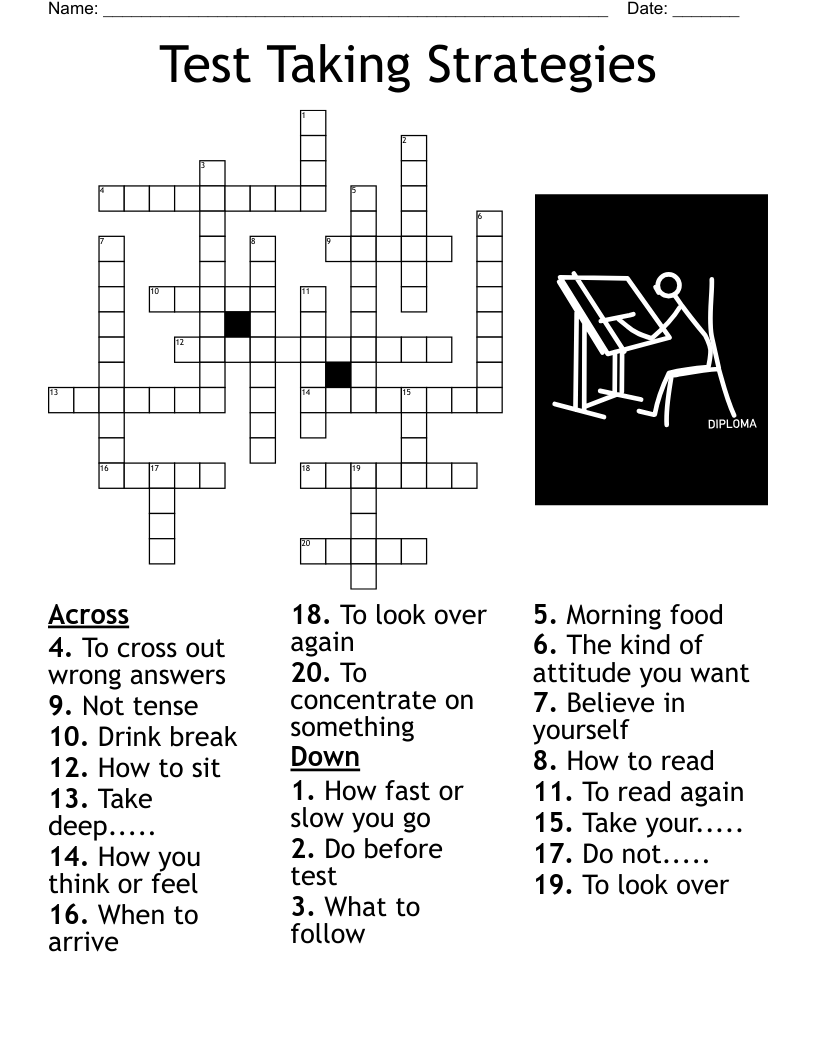
I started with the basics. I knew I needed a way to represent the crossword grid. So, I whipped up a simple 2D array in Python. Each cell could be a letter, a blank space, or a black square (represented by a ‘#’).
Then came the hard part: finding words that fit. My initial idea was to load a dictionary of words and iterate through it, checking if each word could fit in a particular spot on the grid. This involved checking the length of the word against the available space, and also checking if the letters matched up with any existing letters in the grid. Seemed straightforward enough.
I wrote a function called can_place_word(grid, word, row, col, direction)
. This function took the grid, the word, the starting row and column, and the direction (either ‘horizontal’ or ‘vertical’) as input. It meticulously checked if the word could be placed without conflicts. Lots of index juggling and edge-case handling! I remember spending a good chunk of time debugging off-by-one errors here.
Next, I implemented the core solving logic. I made a recursive function called solve_crossword(grid, dictionary)
. It scanned the grid for empty spaces, and for each empty space, it tried to place words from the dictionary using the can_place_word
function. If a word could be placed, it temporarily updated the grid and recursively called solve_crossword
to continue filling the rest of the puzzle. If the recursive call failed (meaning no solution could be found with the current word placement), it would backtrack, remove the word, and try a different one.
The big hurdle was performance. The dictionary I was using was huge, and trying every possible word in every possible location was taking forever. I realized I needed to optimize the word selection process. I added a filtering step to the can_place_word
, only considering words that matched the pattern of known letters in that spot. For instance, if a space had the pattern “_A_E”, it would only consider words with “A” as the second letter and “E” as the fourth. This drastically reduced the number of words the solver had to check.
Another thing I tweaked was the order in which the solver tried to fill the spaces. I started prioritizing filling the spaces with the fewest possible word matches first. This helped to prune the search tree earlier on.
After several hours of coding, debugging, and optimizing, I finally had a working crossword solver! It wasn’t perfect – it still struggled with very complex puzzles – but it could handle simpler ones pretty well. I tested it with a few example crosswords I found online, and it successfully solved them. Seeing the solver fill in the grid automatically was strangely satisfying.
Ultimately, it was a fun and challenging project that reinforced my understanding of recursion, backtracking, and algorithm optimization. Plus, I now have a tool to help me cheat at crosswords! Just kidding… mostly.
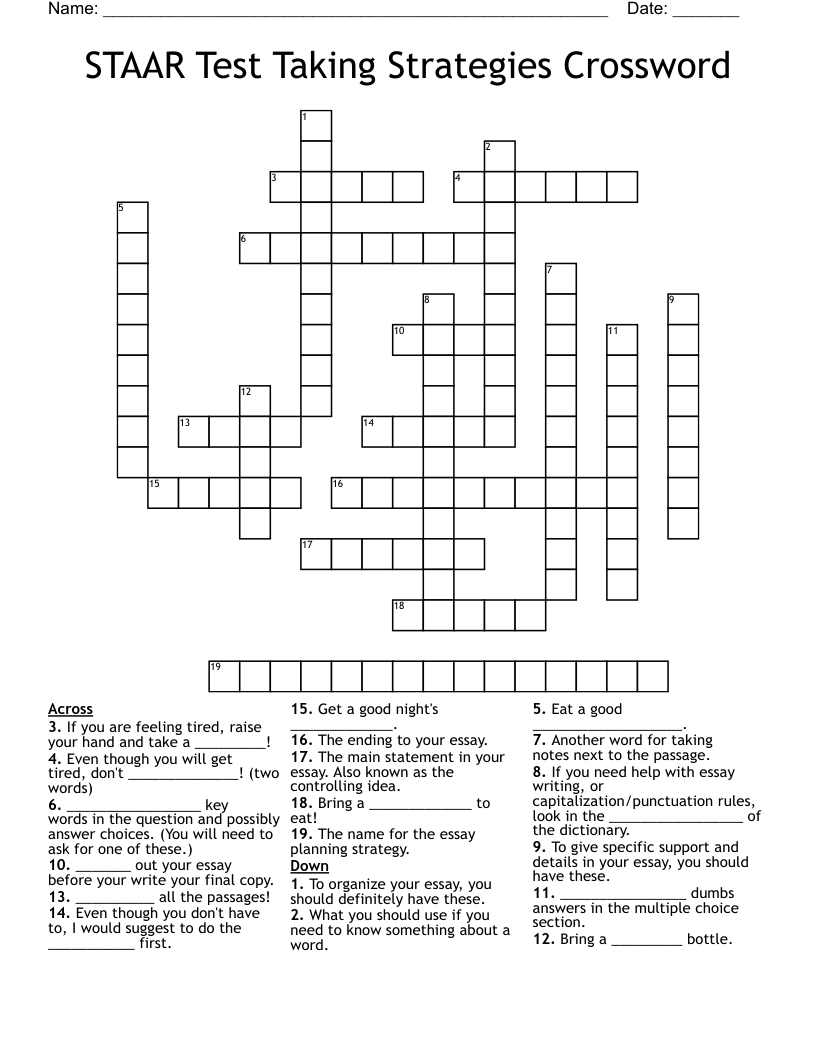